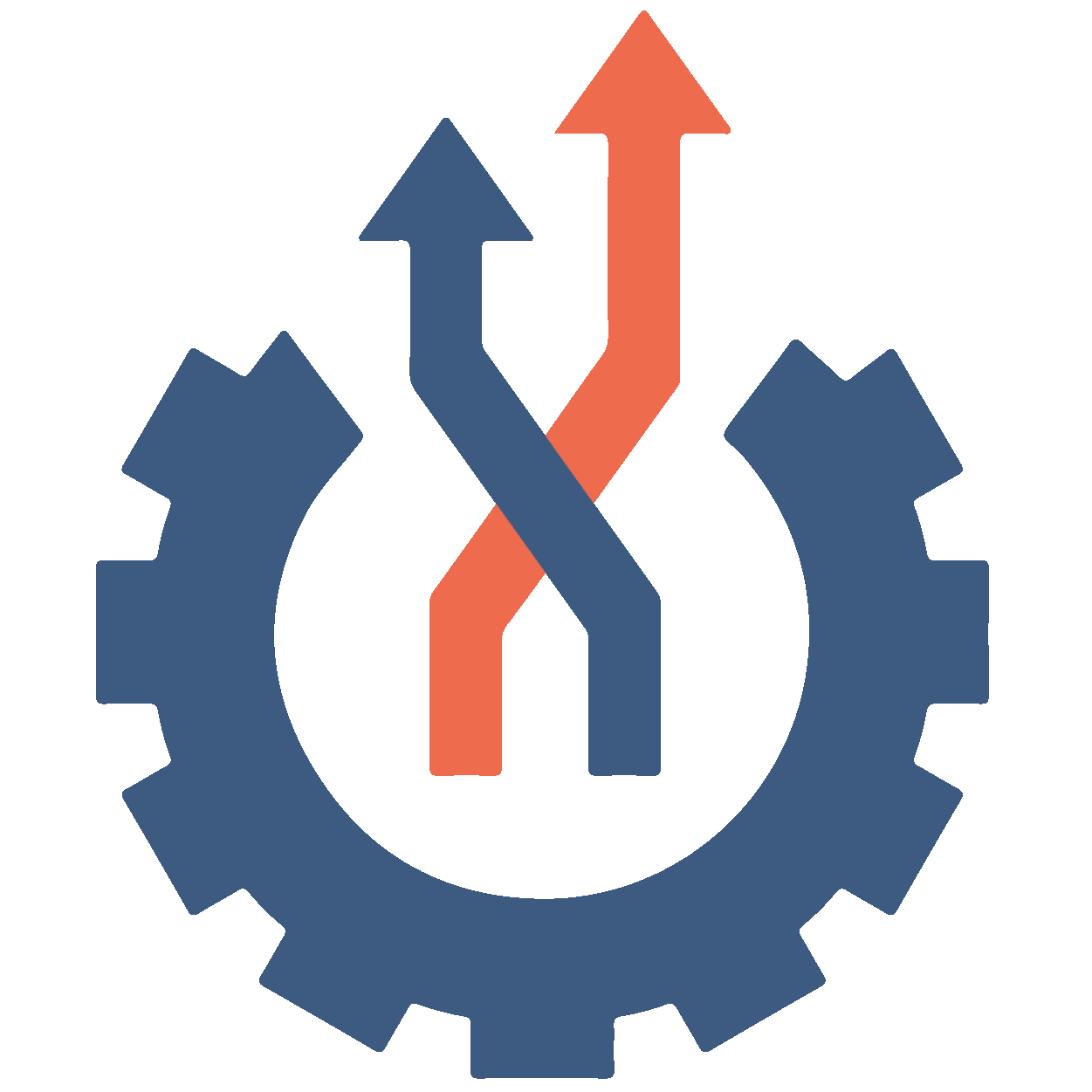
Serverless Workflow
Serverless Workflow presents a vendor-neutral, open-source, and entirely community-driven ecosystem tailored for defining and executing DSL-based workflows in the realm of Serverless technology.
The current version is 1.0.0, get the JSON Schema here: YAML or JSON
Learn MoreThe Serverless Workflow DSL is a high-level language that reshapes the terrain of workflow creation, boasting a design that is ubiquitous, intuitive, imperative, and fluent.
Usability
Designed with linguistic fluency, implicit default behaviors, and minimal technical jargon, making workflows accessible to developers with diverse skill levels and enhancing collaboration.
Event driven
Supports event-driven execution and various scheduling options, including CRON expressions and time-based triggers, to respond efficiently to dynamic conditions.
Interoperability
Seamlessly integrates with multiple protocols (HTTP, gRPC, OpenAPI, AsyncAPI), ensuring easy communication with external systems and services, along with support for custom interactions via scripts, containers, or shell commands.
Platform-Agnostic
Serverless Workflow enables developers to build workflows that can operate across diverse platforms and environments, eliminating the need for platform-specific adaptations.
Extensibility
Provides extensible components and supports defining custom functions and extensions, allowing developers to tailor workflows to unique business requirements without compromising compatibility.
Fault tolerant
Offers comprehensive data transformation, validation, and fault tolerance mechanisms, ensuring workflows are robust, reliable, and capable of handling complex processes and failures gracefully.
User-Friendly DSL: Workflows Made Simple
Async API Example
document:
dsl: '1.0.0'
namespace: default
name: call-asyncapi
version: '1.0.0'
do:
- findPet:
call: asyncapi
with:
document:
uri: https://fake.com/docs/asyncapi.json
operationRef: findPetsByStatus
server: staging
message:
payload:
petId: ${ .pet.id }
authentication:
bearer:
token: ${ .token }
Container Example
document:
dsl: '1.0.0'
namespace: default
name: run-container
version: '1.0.0'
do:
- runContainer:
run:
container:
image: fake-image
Emit Event Example
document:
dsl: '1.0.0'
namespace: default
name: emit
version: '0.1.0'
do:
- emitEvent:
emit:
event:
with:
source: https://petstore.com
type: com.petstore.order.placed.v1
data:
client:
firstName: Cruella
lastName: de Vil
items:
- breed: dalmatian
quantity: 101
For Example
document:
dsl: '1.0.0'
namespace: default
name: for-example
version: '0.1.0'
do:
- checkup:
for:
each: pet
in: .pets
at: index
while: .vet != null
do:
- waitForCheckup:
listen:
to:
one:
with:
type: com.fake.petclinic.pets.checkup.completed.v2
output:
as: '.pets + [{ "id": $pet.id }]'
Fork Example
document:
dsl: '1.0.0'
namespace: default
name: fork-example
version: '0.1.0'
do:
- raiseAlarm:
fork:
compete: true
branches:
- callNurse:
call: http
with:
method: put
endpoint: https://fake-hospital.com/api/v3/alert/nurses
body:
patientId: ${ .patient.fullName }
room: ${ .room.number }
- callDoctor:
call: http
with:
method: put
endpoint: https://fake-hospital.com/api/v3/alert/doctor
body:
patientId: ${ .patient.fullName }
room: ${ .room.number }
gRPC Example
document:
dsl: '1.0.0'
namespace: default
name: call-grpc
version: '1.0.0'
do:
- greet:
call: grpc
with:
proto:
endpoint: file://app/greet.proto
service:
name: GreeterApi.Greeter
host: localhost
port: 5011
method: SayHello
arguments:
name: '${ .user.preferredDisplayName }'
HTTP Example
document:
dsl: '1.0.0'
namespace: default
name: call-http
version: '1.0.0'
do:
- getPet:
call: http
with:
method: get
endpoint: https://petstore.swagger.io/v2/pet/{petId}
Listen Event Example
document:
dsl: '1.0.0'
namespace: default
name: listen-to-all
version: '0.1.0'
do:
- callDoctor:
listen:
to:
all:
- with:
type: com.fake-hospital.vitals.measurements.temperature
data: ${ .temperature > 38 }
- with:
type: com.fake-hospital.vitals.measurements.bpm
data: ${ .bpm < 60 or .bpm > 100 }
Open API Example
document:
dsl: '1.0.0'
namespace: default
name: call-openapi
version: '1.0.0'
do:
- findPet:
call: openapi
with:
document:
endpoint: https://petstore.swagger.io/v2/swagger.json
operationId: findPetsByStatus
parameters:
status: available
Raise Error Example
document:
dsl: '1.0.0'
namespace: default
name: raise-not-implemented
version: '0.1.0'
do:
- notImplemented:
raise:
error:
type: https://serverlessworkflow.io/errors/not-implemented
status: 500
title: Not Implemented
detail: ${ "The workflow '\( $workflow.definition.document.name ):\( $workflow.definition.document.version )' is a work in progress and cannot be run yet" }
Script Example
document:
dsl: '1.0.0'
namespace: samples
name: run-script-with-arguments
version: 0.1.0
do:
- log:
run:
script:
language: javascript
arguments:
message: ${ .message }
code: >
console.log(message)
Subflow Example
document:
dsl: '1.0.0'
namespace: default
name: run-subflow
version: '0.1.0'
do:
- registerCustomer:
run:
workflow:
namespace: default
name: register-customer
version: '0.1.0'
input:
customer: .user
Switch Example
document:
dsl: '1.0.0'
namespace: default
name: switch-example
version: '0.1.0'
do:
- processOrder:
switch:
- case1:
when: .orderType == "electronic"
then: processElectronicOrder
- case2:
when: .orderType == "physical"
then: processPhysicalOrder
- default:
then: handleUnknownOrderType
- processElectronicOrder:
do:
- validatePayment:
call: http
with:
method: post
endpoint: https://fake-payment-service.com/validate
- fulfillOrder:
call: http
with:
method: post
endpoint: https://fake-fulfillment-service.com/fulfill
then: exit
- processPhysicalOrder:
do:
- checkInventory:
call: http
with:
method: get
endpoint: https://fake-inventory-service.com/inventory
- packItems:
call: http
with:
method: post
endpoint: https://fake-packaging-service.com/pack
- scheduleShipping:
call: http
with:
method: post
endpoint: https://fake-shipping-service.com/schedule
then: exit
- handleUnknownOrderType:
do:
- logWarning:
call: http
with:
method: post
endpoint: https://fake-logging-service.com/warn
- notifyAdmin:
call: http
with:
method: post
endpoint: https://fake-notification-service.com/notify
Try-Catch Example
document:
dsl: '1.0.0'
namespace: default
name: try-catch
version: '0.1.0'
do:
- tryGetPet:
try:
- getPet:
call: http
with:
method: get
endpoint: https://petstore.swagger.io/v2/pet/{petId}
catch:
errors:
with:
type: https://serverlessworkflow.io/spec/1.0.0/errors/communication
status: 404
as: error
do:
- notifySupport:
emit:
event:
with:
source: https://petstore.swagger.io
type: io.swagger.petstore.events.pets.not-found.v1
data: ${ $error }
- setError:
set:
error: $error
export:
as: '$context + { error: $error }'
- buyPet:
if: $context.error == null
call: http
with:
method: put
endpoint: https://petstore.swagger.io/v2/pet/{petId}
body: '${ . + { status: "sold" } }'
Wait Example
document:
dsl: '1.0.0'
namespace: default
name: wait-duration-inline
version: '0.1.0'
do:
- wait30Seconds:
wait:
seconds: 30
Visit our GitHub repository for more examples or complete use cases.
Reach out to us!
Join our Meetings!
Add the schedule to your calendar and become a part of our discussions!
How To JoinContributions welcome!
We do a Pull Request contributions workflow on GitHub. New users are always welcome!
To RepositoryJoin us on Slack!
Chat with our community and follow announcements at #serverless-workflow
Open SlackOpen Source projects supporting our DSL
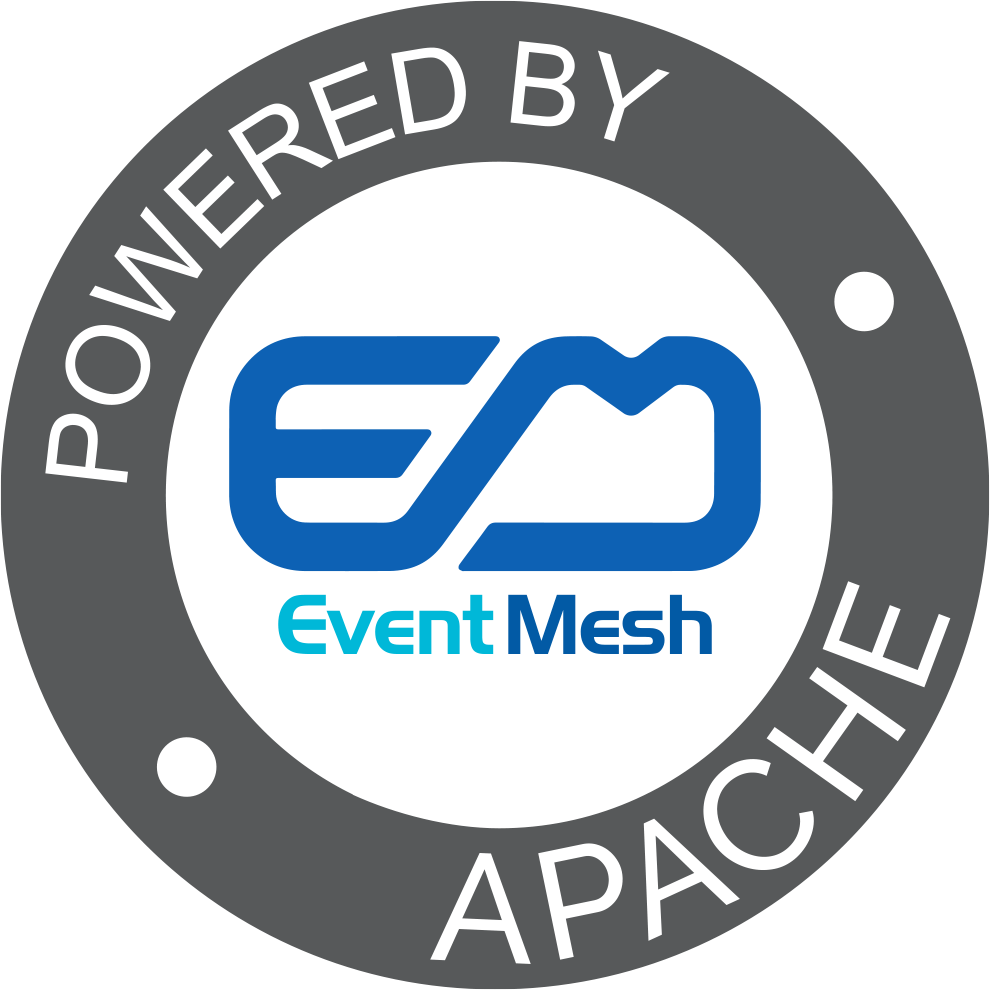
Apache EventMesh Workflow
Apache EventMesh Workflow is a cloud vendor-independent, cloud-native-oriented Serverless Workflow Runtime based on Serverless Workflow specification, and provides durability, reliability, scalability, and observability capabilities.
Get Started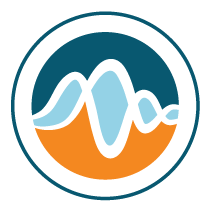
Apache KIE SonataFlow
SonataFlow is a powerful tool for building cloud-native workflow applications, enabling seamless orchestration and choreography of services and events.
Get Started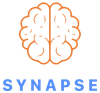
Synapse
Synapse is a vendor-neutral, free, open-source, and community-driven Workflow Management System (WFMS) implementing the Serverless Workflow specification. You can deploy Synapse on Docker, Kubernetes, or natively on Windows, Mac, and Linux.
Get StartedTrusted by top brands in workflow technologies
Already using Serverless Workflow? Join our list of top brands by letting us know here!
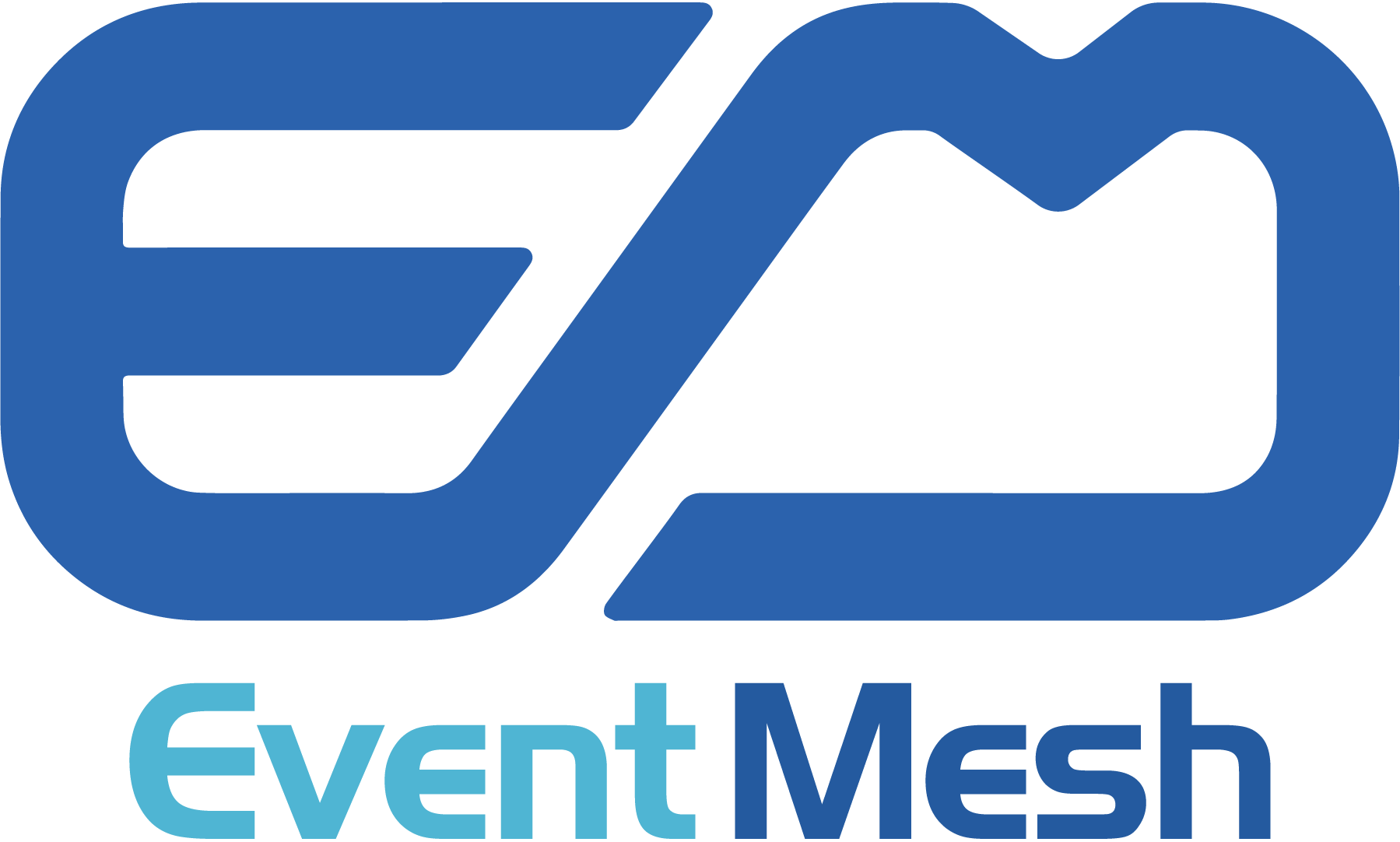
Apache EventMesh
A new generation serverless event middleware for building distributed event-driven applications.
Learn More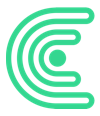
Caf
Serverless Workflow is the core technology behind every KYC/KYB solution allowing them to customize it for their clients seamlessly.
Learn More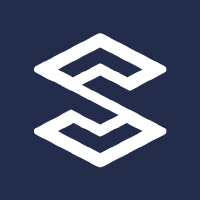
FaasNet
FaasNet makes it easy to deploy functions and API to Kubernetes without repetitive, boiler-plate coding.
Learn MoreFoxflow
Foxflow's AI agent development platform accelerates business process automation and software delivery through LLM-integrated declarative workflows
Learn More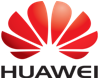
Huawei
Huawei FunctionGraph hosts event-driven functions in a serverless context while ensuring high availability, high scalability, and zero maintenance.
Learn More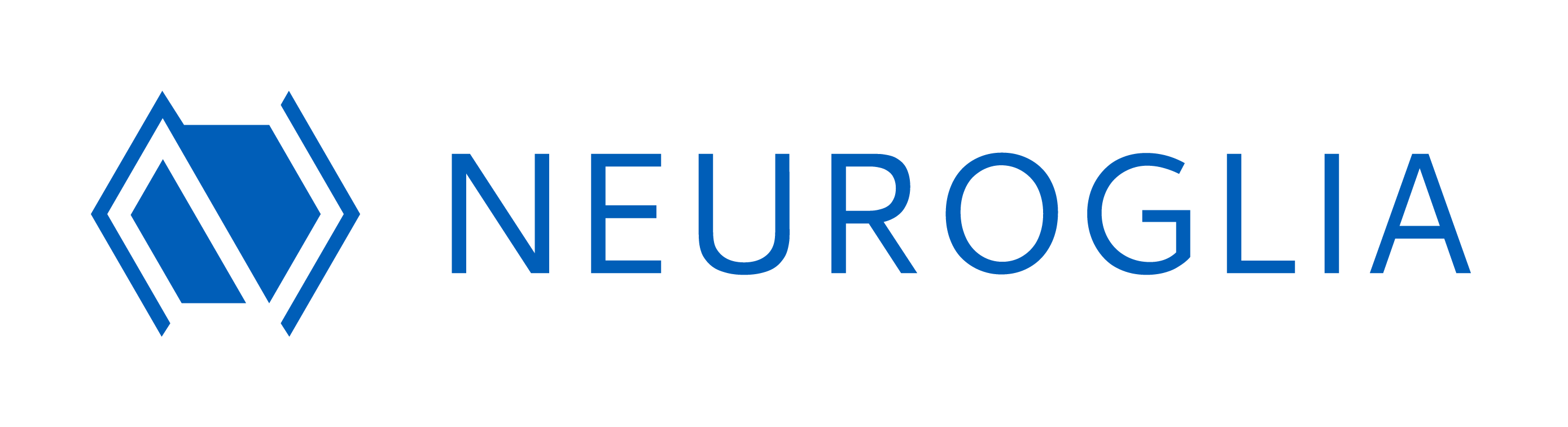
Neuroglia
Neuroglia is a consultancy and solution design company for the digital transformation of companies and their services.
Learn MoreRed Hat
Red Hat sponsors Apache KIE SonataFlow, a tool for creating cloud-native workflows. SonataFlow supports service and event orchestration, integrating with your architecture using CloudEvents, REST calls, and other standard components.
Learn More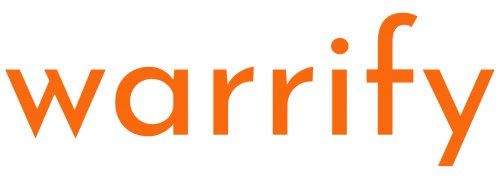
Warrify
Warrify offers a leading data platform for the retail industry. With "warrify Journeys" (powered by Serverless Workflows) retailers are discovering new ways how to engage their customers in real time.
Learn MoreSupport our Project
Our sponsors, along with our community, help our project grow and stay vendor-neutral through their donations.
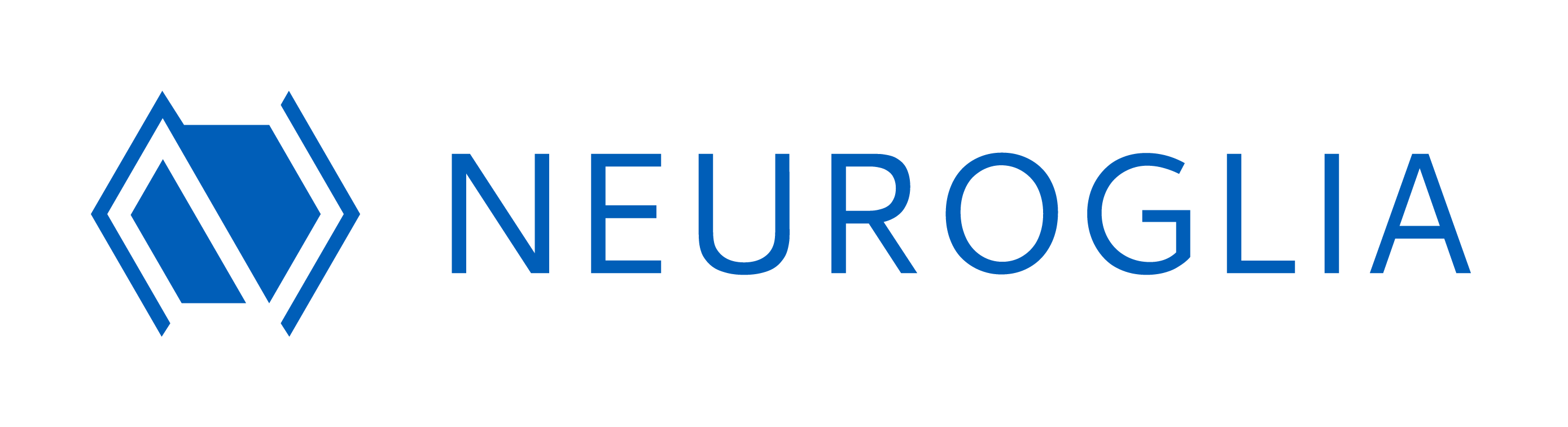
Neuroglia
Neuroglia is a consultancy and solution design company for the digital transformation of companies and their services.
Learn MoreInnovation in the Open
Serverless Workflow is an open-source project under the governance of the Cloud Native Computing Foundation (CNCF) and a part of the Linux Foundation.
The project is in the Application Definition & Image Build landscape.